Section Header Listview in android
Today we are learning about the section header in android.
Here I am using the adapter class for loading the view of list one by one by using get view method.
So lets start the coding about the section header.
Listview
are commonly used to display a large set of similar data. But in the section header list we can show the similar data list in the different and separate section of the list view.Here I am using the adapter class for loading the view of list one by one by using get view method.
So lets start the coding about the section header.
layout/main.xml
1
2
3
4
5
6
7
| <linearlayout android:layout_height= "fill_parent" android:layout_width= "fill_parent" android:orientation= "vertical" xmlns:android= "http://schemas.android.com/apk/res/android" > <textview android:layout_height= "wrap_content" android:layout_width= "fill_parent" android:text= "@string/hello" > <listview android:id= "@+id/listView_main" android:layout_height= "wrap_content" android:layout_width= "match_parent" > </listview> </textview></linearlayout> |
layout/list_item_section.xml
1
2
3
4
5
| <linearlayout android:layout_height= "wrap_content" android:layout_width= "fill_parent" android:orientation= "vertical" xmlns:android= "http://schemas.android.com/apk/res/android" > <include android:id= "@+id/list_item_section_text" layout= "@android:layout/preference_category" > </include></linearlayout> |
layout/list_item_enty.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
| <linearlayout android:gravity= "center_vertical" android:layout_height= "wrap_content" android:layout_width= "fill_parent" android:minheight= "?android:attr/listPreferredItemHeight" android:paddingright= "?android:attr/scrollbarSize" xmlns:android= "http://schemas.android.com/apk/res/android" > <imageview android:id= "@+id/list_item_entry_drawable" android:layout_height= "fill_parent" android:layout_width= "wrap_content" android:paddingleft= "9dp" android:src= "@android:drawable/ic_menu_preferences" > <relativelayout android:layout_height= "wrap_content" android:layout_marginbottom= "6dip" android:layout_marginleft= "10dip" android:layout_marginright= "6dip" android:layout_margintop= "6dip" android:layout_weight= "1" android:layout_width= "wrap_content" > <textview android:ellipsize= "marquee" android:fadingedge= "horizontal" android:id= "@+id/list_item_entry_title" android:layout_height= "wrap_content" android:layout_width= "wrap_content" android:singleline= "true" android:textappearance= "?android:attr/textAppearanceLarge" > <textview android:id= "@+id/list_item_entry_summary" android:layout_alignleft= "@id/list_item_entry_title" android:layout_below= "@id/list_item_entry_title" android:layout_height= "wrap_content" android:layout_width= "wrap_content" android:singleline= "true" android:textappearance= "?android:attr/textAppearanceSmall" android:textcolor= "?android:attr/textColorSecondary" > </textview></textview></relativelayout> </imageview></linearlayout> |
Item.java
1
2
3
4
5
6
7
| package com.sunil.util; public interface Item { public boolean isSection(); } |
SectionItem.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
| package com.sunil.util; public class SectionItem implements Item{ private final String title; public SectionItem(String title) { this .title = title; } public String getTitle(){ return title; } @Override public boolean isSection() { return true ; } } |
EntryItem.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
| package com.sunil.util; public class EntryItem implements Item{ public final String title; public final String subtitle; public EntryItem(String title, String subtitle) { this .title = title; this .subtitle = subtitle; } @Override public boolean isSection() { return false ; } } |
EntryAdapter.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
| package com.sunil.util; import java.util.ArrayList; import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ArrayAdapter; import android.widget.TextView; import com.sunil.sectionlist.R; public class EntryAdapter extends ArrayAdapter<item> { private Context context; private ArrayList<item> items; private LayoutInflater vi; public EntryAdapter(Context context,ArrayList<item> items) { super (context,0, items); this .context = context; this .items = items; vi = (LayoutInflater)context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); } @Override public View getView(int position, View convertView, ViewGroup parent) { View v = convertView; final Item i = items.get(position); if (i != null ) { if (i.isSection()){ SectionItem si = (SectionItem)i; v = vi.inflate(R.layout.list_item_section, null ); v.setOnClickListener( null ); v.setOnLongClickListener( null ); v.setLongClickable( false ); final TextView sectionView = (TextView) v.findViewById(R.id.list_item_section_text); sectionView.setText(si.getTitle()); } else { EntryItem ei = (EntryItem)i; v = vi.inflate(R.layout.list_item_entry, null ); final TextView title = (TextView)v.findViewById(R.id.list_item_entry_title); final TextView subtitle = (TextView)v.findViewById(R.id.list_item_entry_summary); if (title != null ) title.setText(ei.title); if (subtitle != null ) subtitle.setText(ei.subtitle); } } return v; } } </item></item></item> |
SectionListExampleActivity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
| package com.sunil.sectionactivity; import java.util.ArrayList; import com.sunil.util.EntryAdapter; import com.sunil.util.EntryItem; import com.sunil.util.Item; import com.sunil.util.SectionItem; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ListView; import android.widget.Toast; import com.sunil.sectionlist.R; public class SectionListExampleActivity extends Activity implements OnItemClickListener{ ArrayList<item> items = new ArrayList<item>(); ListView listview= null ; @Override public void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.main); listview=(ListView)findViewById(R.id.listView_main); items.add( new SectionItem( "My Friends" )); items.add( new EntryItem( "Abhi Tripathi" , "Champpu" )); items.add( new EntryItem( "Sandeep Pal" , "Sandy kaliya" )); items.add( new EntryItem( "Amit Verma" , "Budhiya" )); items.add( new EntryItem( "Awadhesh Diwaker " , "Dadda" )); items.add( new SectionItem( "Android Version" )); items.add( new EntryItem( "Jelly Bean" , "android 4.2" )); items.add( new EntryItem( "IceCream Sandwich" , "android 4.0" )); items.add( new EntryItem( "Honey Comb" , "android 3.0" )); items.add( new EntryItem( "Ginger Bread " , "android 2.2" )); items.add( new SectionItem( "Android Phones" )); items.add( new EntryItem( "Samsung" , "Gallexy" )); items.add( new EntryItem( "Sony Ericson" , "Xperia" )); items.add( new EntryItem( "Nokiya" , "Lumia" )); EntryAdapter adapter = new EntryAdapter( this , items); listview.setAdapter(adapter); listview.setOnItemClickListener( this ); } @Override public void onItemClick(AdapterView arg0, View arg1, int position, long arg3) { EntryItem item = (EntryItem)items.get(position); Toast.makeText( this , "You clicked " + item.title , Toast.LENGTH_SHORT).show(); } } </item></item> |
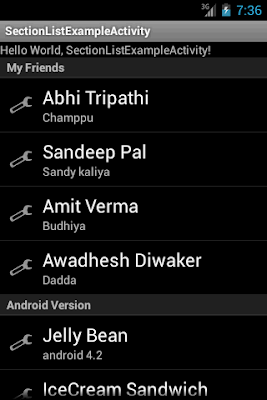
Comments
Post a Comment